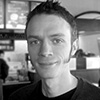
Here’s a quick PowerShell tip…
TL;DR
Have you ever wanted to get a list of running desktop applications in PowerShell? Maybe you want to see what you have open but would rather not fumble around with Task Manager?
Here’s a one-liner to do just that:
gps | ? { $_.MainWindowTitle }
Sample output:
Handles NPM(K) PM(K) WS(K) CPU(s) Id SI ProcessName
------- ------ ----- ----- ------ -- -- -----------
4269 188 382940 424100 1,336.84 12888 1 chrome
37 3 1568 2560 0.05 5176 1 cmd
927 54 53892 69800 8.05 8988 1 EXCEL
1385 105 87956 98928 157.45 5024 1 explorer
212 15 26572 18204 16.58 13256 1 gvim
Breaking it Down
The first command, gps
, is a built-in alias for Get-Process
, and ?
is an alias for Where-Object
. So, by specifying the MainWindowTitle
property, we’re essentially limiting our output to only those processes that have window titles.
If we were to expand the previous command line into full cmdlet names, it would look like this:
Get-Process | Where-Object { $_.MainWindowTitle }
List Running Apps with their Titles and Process IDs
Now let’s add a little more to the previous command line in order make it a bit more useful to us.
Get-Process | Where-Object { $_.MainWindowTitle } | Format-Table ID,Name,Mainwindowtitle –AutoSize
Here some sample output:
Id Name MainWindowTitle
-- ---- ---------------
6040 ApplicationFrameHost Windows Defender Security Center
5036 cmd Command Prompt
5148 powershell Windows PowerShell
This gives us the window title, so that we know what to look for in the task bar; it gives us the process names, so that we know what executable is running “underneath” those window titles; and it gives us the process ID, so that we can work with a specific process easily if we need to.
We can put all this into a function in our PowerShell Profile and have it to use whenever we need it:
function Get-DesktopApps {
Get-Process | Where-Object { $_.MainWindowTitle } |
Format-Table ID,Name,Mainwindowtitle –AutoSize
}
Additional Reading
- Documentation: Get-Process
- Documentation: Where-Object
- Documentation: Format-Table