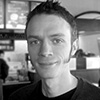
In this post, I’m going to walk through how to expand a managed OS disk on an Azure virtual machine. In subsequent posts, we will cover how to convert this into a script for use interactively or with automation tools.
Log In and Verify Subscription
I always like to include a reminder to validate that you are logged in to the correct Azure Subscription. For those of us with multiple clients and subscriptions to manage, failure to do this can have serious consequences, so I suggest do so every time, and building some kind of verification logic into your automation scripts as well, where it makes sense.
az account list -o table
Under the IsDefault
column, make sure that you see True
next to the subscription you are intending to work with.
Deallocate the Virtual Machine
In order to resize virtual hard disks, we have to deallocate the virtual machine first, because as of this writing resizing them on-the-fly is not possible.
az vm deallocate \
--resource-group $rg \
--name $vm
In this example, and throughout the post, I refer to variables instead of the literal names. Using variables makes it easier to convert this to a script later and it allows you to use the examples on whatever specific VM you wish if you’re following along.
We are doing our work in Bash, so to use these variables names we simply assign them values, as in this example:
rg=lab1-rg
vm=ubuntuserver1
If we want to verify our VM is deallocated, we can use this query, which filters the output on the virtual machine name, and outputs the name along with the power state:
az vm list \
--resource-group $rg \
--query "[?name == '$vm'].{Name:name, PowerState:powerState}" \
--show-details \
--output table
Example output:
Name PowerState
----------- --------------
ubuntutest1 VM deallocated
Note that in the command above we had to include the --show-details
switch in order to have the powerState
property returned in the output. Otherwise, that column would have been left off.
Get the Current Disk Size
Next we should determine the current size of the disk, so we can compare it against the size we want to expand it to. The first step is to locate the disk ID and assign it to a variable, with the following query:
disk_id=$(az vm list -g $rg \
--query "[?name == '$vm'].storageProfile.osDisk.managedDisk.id" \
--output tsv)
Now that we have the disk ID assigned to $disk_id
, we can use it to lookup the disk:
az disk list \
--resource-group $rg \
--query "[?(id == '$disk_id')].{Name:name,Gb:diskSizeGb,Tier:sku.name}" \
--output table
Example output:
$ az disk list \
> --resource-group $rg \
> --query "[?(id == '$disk_id')].{Name:name,Gb:diskSizeGb,Tier:sku.name}" \
> --output table
Name Gb Tier
------------------- ---- ------------
ubuntutest1-disk-os 30 Standard_LRS
This is good output because it confirms both the current size of the disk, and reminds us what storage tier it’s using, in case that’s a concern.
Aside: If you’re inquisitive, you may be asking why we didn’t just look up the disk directly, by name. We could have. The reason we didn’t is that most of the time we need to expand a disk, we are doing so based on some request that refers to the virtual machine by name, not the disk. Additionally, in some environments disk names are “square” with the virtual machine names, and in others, they aren’t. So, it’s a good idea to start at the source and programmatically move to the destination.
Next, we need to store the current disk size to compare it against the requested size:
disk_size_new=50
disk_size=$(az disk list -g $rg \
--query "[?(id == '$disk_id')].diskSizeGb" \
--output tsv)
(Ordinarily we wouldn’t break those lines up, but doing so makes it easier to read.)
Compare Current Disk Size to Requested Size
Now that we have the current disk size assigned to $disk_size
, we can verify that the requested size is greater than the current size:
if ! expr $disk_size_new \> $disk_size > /dev/null
then
echo ERROR: Current disk size $disk_size GB is equal to \
or larger than the requested size of $disk_size_new GB.
else
echo "Make it so."
fi
What we’re doing is using the coreutil expr
(short for “expression”) to evaluate whether $disk_size_new
is greater than $disk_size
(the current size of the disk). You’ll notice that instead of just using the greater-than carot here, we’ve had to escape it with a backslash: \>
. The bang (!
) indicates NOT
, so the first condition is satisfied if the requested size is not larger than the existing. And of course, where I’ve got messages, you can do whatever you want with the conditions — exit the script, write an error, send an alert, etc.
Update the Disk Size
Now, without any further ado, let’s expand the disk:
disk_size_new=50
az disk update \
--resource-group $rg \
--ids $disk_id \
--size-gb $disk_size_new
An alternative way to do this is by the disk name:
disk_size_new=50
disk_name=$(az disk list -g $rg --query "[?(id == '$disk_id')].name" -o tsv)
az disk update \
--resource-group $rg \
--name $disk_name \
--size-gb $disk_size_new \
--output table
Verify Final Disk Size
And then we verify our results:
az disk list \
--resource-group $rg \
--query "[?name == '$disk_name'].{Name:name, DiskSize:diskSizeGb}"
Example output:
Name DiskSize
------------------- ----------
ubuntutest1-disk-os 50
Start Up the Virtual Machine
All that remains is to boot up the VM:
az vm start -g $rg -n $vm
That’s it! I hope that was helpful and informative. In the next post, we’ll explore how to do disk expansions with PowerShell!
Change Log
2018-07-26
- Added subheadings for easier reading and a reminder about checking subscriptions prior to starting.
Additional Reading
- Documentation: Expand virtual hard disks on a Linux VM in Azure
- Documentation: Expand the OS drive of a Windows VM in an Azure